The configuration option mode
, also available as query param, can be used to select different display modes.
The following display modes are supported:
full
This is the default mode with toolbars, editor and result panes.
Example: https://livecodes.io/?template=react
Screenshot: (App in full mode)
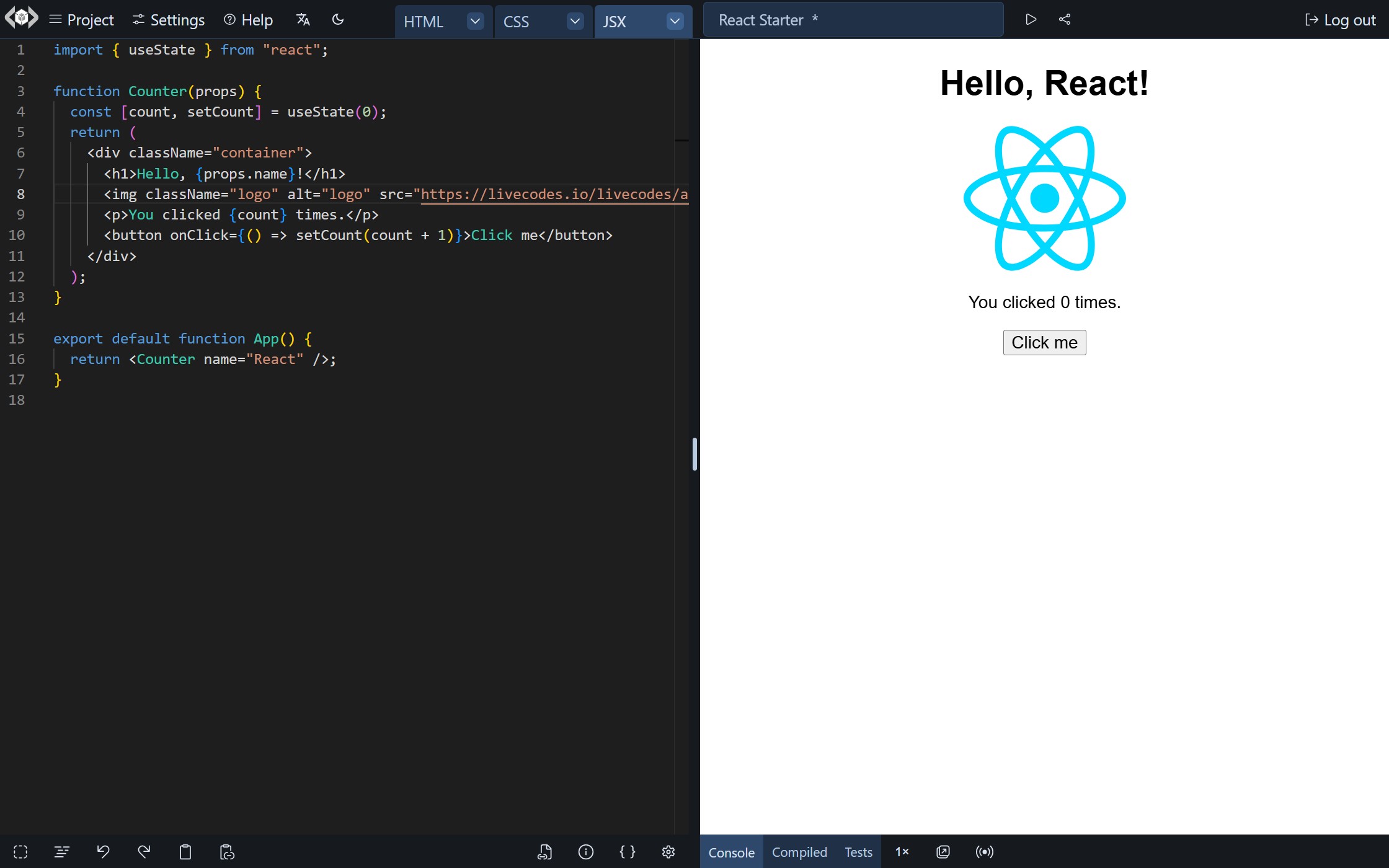
Demo: (Embedded playground in full mode)
show code
import { createPlayground } from 'livecodes';
const options = {
"template": "react"
};
createPlayground('#container', options);
import { createPlayground, type EmbedOptions } from 'livecodes';
const options: EmbedOptions = {
"template": "react"
};
createPlayground('#container', options);
import LiveCodes from 'livecodes/react';
export default function App() {
const options = {
"template": "react"
};
return (<LiveCodes {...options}></LiveCodes>);
}
<script setup>
import LiveCodes from "livecodes/vue";
const options = {
"template": "react"
};
</script>
<template>
<LiveCodes v-bind="options" />
</template>
<script>
import { onMount } from 'svelte';
import { createPlayground } from 'livecodes';
let options = $state({
"template": "react"
});
let container = $state(null);
onMount(() => {
createPlayground(container, options);
});
</script>
<div bind:this="{container}"></div>
focus
This hides most of UI buttons and menus and keeps only the essential elements: editors, editor titles, result page, console, and run and share buttons. It can be toggled during runtime from the full mode through the UI from a button in the lower left corner. Also the query param ?mode=focus
.
Example: https://livecodes.io/?template=react&mode=focus
Screenshot: (focus mode)
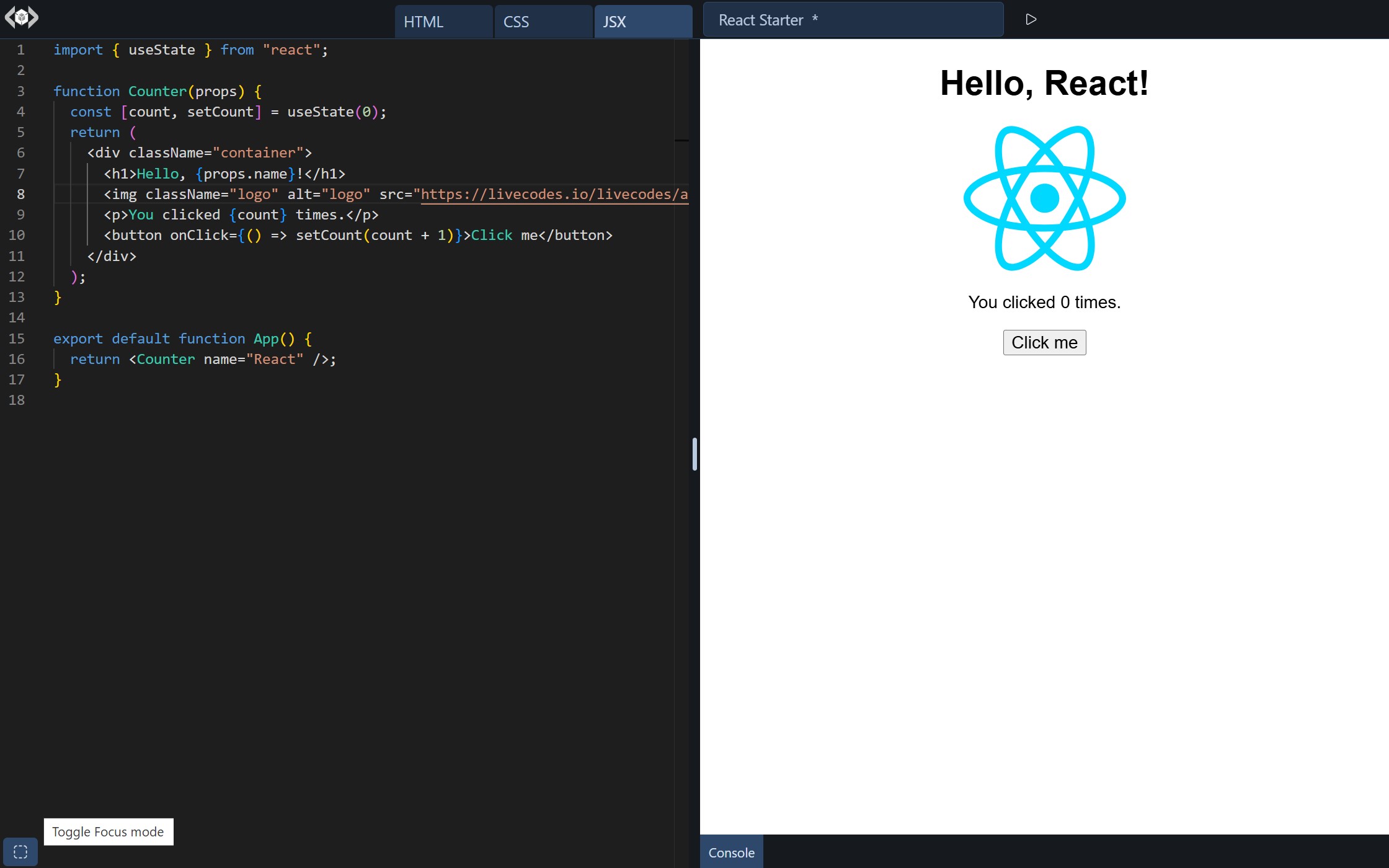
simple
This mode is mainly useful for embedded playgrounds.
It shows only 1 editor with the output (result page +/- console). The content of other editors can be set using SDK config even though the editors are not shown.
By default, codemirror
editor is used, however, this can be changed by the editor
option.
By default, the layout is responsive
but can also be overridden by the layout
option to vertical
or horizontal
.
Demo: JS with console
show code
import { createPlayground } from 'livecodes';
const options = {
"params": {
"mode": "simple",
"js": "console.log(\"hello world\")",
"layout": "vertical",
"console": "full"
}
};
createPlayground('#container', options);
import { createPlayground, type EmbedOptions } from 'livecodes';
const options: EmbedOptions = {
"params": {
"mode": "simple",
"js": "console.log(\"hello world\")",
"layout": "vertical",
"console": "full"
}
};
createPlayground('#container', options);
import LiveCodes from 'livecodes/react';
export default function App() {
const options = {
"params": {
"mode": "simple",
"js": "console.log(\"hello world\")",
"layout": "vertical",
"console": "full"
}
};
return (<LiveCodes {...options}></LiveCodes>);
}
<script setup>
import LiveCodes from "livecodes/vue";
const options = {
"params": {
"mode": "simple",
"js": "console.log(\"hello world\")",
"layout": "vertical",
"console": "full"
}
};
</script>
<template>
<LiveCodes v-bind="options" />
</template>
<script>
import { onMount } from 'svelte';
import { createPlayground } from 'livecodes';
let options = $state({
"params": {
"mode": "simple",
"js": "console.log(\"hello world\")",
"layout": "vertical",
"console": "full"
}
});
let container = $state(null);
onMount(() => {
createPlayground(container, options);
});
</script>
<div bind:this="{container}"></div>
Demo: JSX & Result page (Monaco editor, add CSS)
show code
import { createPlayground } from 'livecodes';
const options = {
"config": {
"mode": "simple",
"layout": "vertical",
"activeEditor": "script",
"editor": "monaco",
"tools": {
"status": "none"
},
"script": {
"language": "jsx",
"content": "import { atom, useAtom } from 'jotai';\n\nconst countAtom = atom(0);\n\nconst Counter = () => {\n const [count, setCount] = useAtom(countAtom);\n const inc = () => setCount((c) => c + 1);\n return (\n <>\n {count} <button onClick={inc}>+1</button>\n </>\n );\n};\n\nconst App = () => (\n <div className=\"App\">\n <h1>Hello Jotai</h1>\n <h2>Enjoy coding!</h2>\n <Counter />\n </div>\n);\n\nexport default App;\n"
},
"style": {
"language": "css",
"content": ".App {\n font-family: sans-serif;\n text-align: center;\n}\n"
}
}
};
createPlayground('#container', options);
import { createPlayground, type EmbedOptions } from 'livecodes';
const options: EmbedOptions = {
"config": {
"mode": "simple",
"layout": "vertical",
"activeEditor": "script",
"editor": "monaco",
"tools": {
"status": "none"
},
"script": {
"language": "jsx",
"content": "import { atom, useAtom } from 'jotai';\n\nconst countAtom = atom(0);\n\nconst Counter = () => {\n const [count, setCount] = useAtom(countAtom);\n const inc = () => setCount((c) => c + 1);\n return (\n <>\n {count} <button onClick={inc}>+1</button>\n </>\n );\n};\n\nconst App = () => (\n <div className=\"App\">\n <h1>Hello Jotai</h1>\n <h2>Enjoy coding!</h2>\n <Counter />\n </div>\n);\n\nexport default App;\n"
},
"style": {
"language": "css",
"content": ".App {\n font-family: sans-serif;\n text-align: center;\n}\n"
}
}
};
createPlayground('#container', options);
import LiveCodes from 'livecodes/react';
export default function App() {
const options = {
"config": {
"mode": "simple",
"layout": "vertical",
"activeEditor": "script",
"editor": "monaco",
"tools": {
"status": "none"
},
"script": {
"language": "jsx",
"content": "import { atom, useAtom } from 'jotai';\n\nconst countAtom = atom(0);\n\nconst Counter = () => {\n const [count, setCount] = useAtom(countAtom);\n const inc = () => setCount((c) => c + 1);\n return (\n <>\n {count} <button onClick={inc}>+1</button>\n </>\n );\n};\n\nconst App = () => (\n <div className=\"App\">\n <h1>Hello Jotai</h1>\n <h2>Enjoy coding!</h2>\n <Counter />\n </div>\n);\n\nexport default App;\n"
},
"style": {
"language": "css",
"content": ".App {\n font-family: sans-serif;\n text-align: center;\n}\n"
}
}
};
return (<LiveCodes {...options}></LiveCodes>);
}
<script setup>
import LiveCodes from "livecodes/vue";
const options = {
"config": {
"mode": "simple",
"layout": "vertical",
"activeEditor": "script",
"editor": "monaco",
"tools": {
"status": "none"
},
"script": {
"language": "jsx",
"content": "import { atom, useAtom } from 'jotai';\n\nconst countAtom = atom(0);\n\nconst Counter = () => {\n const [count, setCount] = useAtom(countAtom);\n const inc = () => setCount((c) => c + 1);\n return (\n <>\n {count} <button onClick={inc}>+1</button>\n </>\n );\n};\n\nconst App = () => (\n <div className=\"App\">\n <h1>Hello Jotai</h1>\n <h2>Enjoy coding!</h2>\n <Counter />\n </div>\n);\n\nexport default App;\n"
},
"style": {
"language": "css",
"content": ".App {\n font-family: sans-serif;\n text-align: center;\n}\n"
}
}
};
</script>
<template>
<LiveCodes v-bind="options" />
</template>
<script>
import { onMount } from 'svelte';
import { createPlayground } from 'livecodes';
let options = $state({
"config": {
"mode": "simple",
"layout": "vertical",
"activeEditor": "script",
"editor": "monaco",
"tools": {
"status": "none"
},
"script": {
"language": "jsx",
"content": "import { atom, useAtom } from 'jotai';\n\nconst countAtom = atom(0);\n\nconst Counter = () => {\n const [count, setCount] = useAtom(countAtom);\n const inc = () => setCount((c) => c + 1);\n return (\n <>\n {count} <button onClick={inc}>+1</button>\n </>\n );\n};\n\nconst App = () => (\n <div className=\"App\">\n <h1>Hello Jotai</h1>\n <h2>Enjoy coding!</h2>\n <Counter />\n </div>\n);\n\nexport default App;\n"
},
"style": {
"language": "css",
"content": ".App {\n font-family: sans-serif;\n text-align: center;\n}\n"
}
}
});
let container = $state(null);
onMount(() => {
createPlayground(container, options);
});
</script>
<div bind:this="{container}"></div>
lite
Loads a light-weight, minimal code editor, with limited playground features.
See the section about lite mode for details
Example: https://livecodes.io/?mode=lite&template=react
Demo:
show code
import { createPlayground } from 'livecodes';
const options = {
"config": {
"mode": "lite"
},
"template": "react"
};
createPlayground('#container', options);
import { createPlayground, type EmbedOptions } from 'livecodes';
const options: EmbedOptions = {
"config": {
"mode": "lite"
},
"template": "react"
};
createPlayground('#container', options);
import LiveCodes from 'livecodes/react';
export default function App() {
const options = {
"config": {
"mode": "lite"
},
"template": "react"
};
return (<LiveCodes {...options}></LiveCodes>);
}
<script setup>
import LiveCodes from "livecodes/vue";
const options = {
"config": {
"mode": "lite"
},
"template": "react"
};
</script>
<template>
<LiveCodes v-bind="options" />
</template>
<script>
import { onMount } from 'svelte';
import { createPlayground } from 'livecodes';
let options = $state({
"config": {
"mode": "lite"
},
"template": "react"
});
let container = $state(null);
onMount(() => {
createPlayground(container, options);
});
</script>
<div bind:this="{container}"></div>
editor
Hides the results pane and works as editor only.
Example: https://livecodes.io/?mode=editor&template=react
Demo:
show code
import { createPlayground } from 'livecodes';
const options = {
"config": {
"mode": "editor"
},
"template": "react"
};
createPlayground('#container', options);
import { createPlayground, type EmbedOptions } from 'livecodes';
const options: EmbedOptions = {
"config": {
"mode": "editor"
},
"template": "react"
};
createPlayground('#container', options);
import LiveCodes from 'livecodes/react';
export default function App() {
const options = {
"config": {
"mode": "editor"
},
"template": "react"
};
return (<LiveCodes {...options}></LiveCodes>);
}
<script setup>
import LiveCodes from "livecodes/vue";
const options = {
"config": {
"mode": "editor"
},
"template": "react"
};
</script>
<template>
<LiveCodes v-bind="options" />
</template>
<script>
import { onMount } from 'svelte';
import { createPlayground } from 'livecodes';
let options = $state({
"config": {
"mode": "editor"
},
"template": "react"
});
let container = $state(null);
onMount(() => {
createPlayground(container, options);
});
</script>
<div bind:this="{container}"></div>
codeblock
A read-only mode showing only the code block without editor interface. On mouse-over a copy button appears that allows to copy the code. This is specially useful when embedded.
Example: https://livecodes.io/?mode=codeblock&template=react
Demo:
show code
import { createPlayground } from 'livecodes';
const options = {
"config": {
"mode": "codeblock"
},
"template": "react"
};
createPlayground('#container', options);
import { createPlayground, type EmbedOptions } from 'livecodes';
const options: EmbedOptions = {
"config": {
"mode": "codeblock"
},
"template": "react"
};
createPlayground('#container', options);
import LiveCodes from 'livecodes/react';
export default function App() {
const options = {
"config": {
"mode": "codeblock"
},
"template": "react"
};
return (<LiveCodes {...options}></LiveCodes>);
}
<script setup>
import LiveCodes from "livecodes/vue";
const options = {
"config": {
"mode": "codeblock"
},
"template": "react"
};
</script>
<template>
<LiveCodes v-bind="options" />
</template>
<script>
import { onMount } from 'svelte';
import { createPlayground } from 'livecodes';
let options = $state({
"config": {
"mode": "codeblock"
},
"template": "react"
});
let container = $state(null);
onMount(() => {
createPlayground(container, options);
});
</script>
<div bind:this="{container}"></div>
By default, in codeblock
mode, the light-weight CodeJar
editor is used (in read-only mode). You can override this by setting the editor
option. Refer to Editor Settings for details.
Example: https://livecodes.io/?mode=codeblock&editor=monaco&template=react
Demo:
show code
import { createPlayground } from 'livecodes';
const options = {
"config": {
"mode": "codeblock",
"editor": "monaco"
},
"template": "react"
};
createPlayground('#container', options);
import { createPlayground, type EmbedOptions } from 'livecodes';
const options: EmbedOptions = {
"config": {
"mode": "codeblock",
"editor": "monaco"
},
"template": "react"
};
createPlayground('#container', options);
import LiveCodes from 'livecodes/react';
export default function App() {
const options = {
"config": {
"mode": "codeblock",
"editor": "monaco"
},
"template": "react"
};
return (<LiveCodes {...options}></LiveCodes>);
}
<script setup>
import LiveCodes from "livecodes/vue";
const options = {
"config": {
"mode": "codeblock",
"editor": "monaco"
},
"template": "react"
};
</script>
<template>
<LiveCodes v-bind="options" />
</template>
<script>
import { onMount } from 'svelte';
import { createPlayground } from 'livecodes';
let options = $state({
"config": {
"mode": "codeblock",
"editor": "monaco"
},
"template": "react"
});
let container = $state(null);
onMount(() => {
createPlayground(container, options);
});
</script>
<div bind:this="{container}"></div>
result
Shows the result page only, with a drawer at the bottom (which can be closed) that allows opening the project in the full playground.
Example: https://livecodes.io/?mode=result&template=react
Demo:
show code
import { createPlayground } from 'livecodes';
const options = {
"params": {
"mode": "result",
"template": "react"
}
};
createPlayground('#container', options);
import { createPlayground, type EmbedOptions } from 'livecodes';
const options: EmbedOptions = {
"params": {
"mode": "result",
"template": "react"
}
};
createPlayground('#container', options);
import LiveCodes from 'livecodes/react';
export default function App() {
const options = {
"params": {
"mode": "result",
"template": "react"
}
};
return (<LiveCodes {...options}></LiveCodes>);
}
<script setup>
import LiveCodes from "livecodes/vue";
const options = {
"params": {
"mode": "result",
"template": "react"
}
};
</script>
<template>
<LiveCodes v-bind="options" />
</template>
<script>
import { onMount } from 'svelte';
import { createPlayground } from 'livecodes';
let options = $state({
"params": {
"mode": "result",
"template": "react"
}
});
let container = $state(null);
onMount(() => {
createPlayground(container, options);
});
</script>
<div bind:this="{container}"></div>
The tools pane (e.g. console/compiled code viewer) is hidden by default in result
mode. It can be shown if set to open
or full
. Refer to Tools pane documentation for details.
Example: https://livecodes.io/?mode=result&tools=console|full&&js=console.log("Hello%20World!")
Demo:
show code
import { createPlayground } from 'livecodes';
const options = {
"params": {
"mode": "result",
"tools": "console|full",
"js": "console.log(\"Hello World!\")"
}
};
createPlayground('#container', options);
import { createPlayground, type EmbedOptions } from 'livecodes';
const options: EmbedOptions = {
"params": {
"mode": "result",
"tools": "console|full",
"js": "console.log(\"Hello World!\")"
}
};
createPlayground('#container', options);
import LiveCodes from 'livecodes/react';
export default function App() {
const options = {
"params": {
"mode": "result",
"tools": "console|full",
"js": "console.log(\"Hello World!\")"
}
};
return (<LiveCodes {...options}></LiveCodes>);
}
<script setup>
import LiveCodes from "livecodes/vue";
const options = {
"params": {
"mode": "result",
"tools": "console|full",
"js": "console.log(\"Hello World!\")"
}
};
</script>
<template>
<LiveCodes v-bind="options" />
</template>
<script>
import { onMount } from 'svelte';
import { createPlayground } from 'livecodes';
let options = $state({
"params": {
"mode": "result",
"tools": "console|full",
"js": "console.log(\"Hello World!\")"
}
});
let container = $state(null);
onMount(() => {
createPlayground(container, options);
});
</script>
<div bind:this="{container}"></div>
Display Mode vs Default View
"Display Mode" is different from "Default View".
In editor
display mode, only the editor is loaded and the result page is not available. While editor
default view shows the editor by default, and the result page can be shown by dragging the split gutter.
The same applies for result
display mode and default view.